The basics of c programming language
C is a powerful language with a massive built-in library facility. You can build a calculator to an operating system, just by learning C and assembly! (Assembly is needed to develop an operating system).
We human beings communicate with others using letters or symbols. But a computer is an electrical device, which knows nothing other than 1 and 0s. Here, 1 means presence of electricity in the motherboard, and 0 means the absence. So, when the computer was used, scientist used this method to communicate with the computer, to give it orders. They generated electricity and again, stopped generating, which created a pattern of 1s and 0s. This can be referred as a language, known as machine language.
The machine language was hard to understand. And scientists faced millions of problems when they were coding using the machine language.
Imagine you have travelled to a country, where people speak in a language you don’t know. They also have no idea about what are you saying. So you need a interpreter, who can convert your language into theirs, and their language into yours. So, scientists created an interpreter, in which we can give commands to a pc using English, and that interpreter will convert your language to the language which computer understands and vice versa. That language was known as the Assembly Language.
Assembly Language was handy, when it came to distinguish the problems scientists were facing during the communication with computers. But it took a lot of time and effort to write the code, the syntax was hard to remember, complex and difficult. It didn’t allow executing the code in another machine, and took more memory when running big programs.
To resolve the problems, scientist later invented the third generation programming languages, which had easy syntax to remember, had the WORA facility (Write once, run anywhere) and used lower memory, which allowed them to execute codes faster. As I said earlier, we need a translator or interpreter which allows us to command the pc using our innate language. Third generation programming languages used complex translators, which were known as compilers.
This picture below can ease the process of compiling a code.
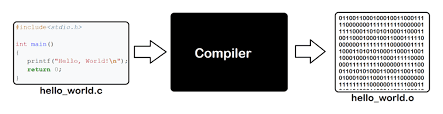
A program was wrote in English, which were translated using a compiler and turned into machine language.
Every language has its fixed words, syntaxes and grammars. So programming languages also own some structures, syntaxes and rules, which are required to execute a program in a machines motherboard. . In this article, we will try to teach you exactly that!
At first, take a look at this program.
# include
<stdio.h>
int main()
{
printf (“Hello
world!”);
return 0;
}
This program will result in…
>>>Hello
world!
in the first line we wrote, # include <stdio.h>. Take a closer look at the code. We wrote some lines in it, like printf (“Hello world!”);, which basically prints the line “hello world!” on the screen. But we know that computer doesn’t know anything other than 1s and 0s. So we use the compiler to translate this command into 1s and 0s, and the compiler speaks in c language, the c language says printf means print anything to the screen. And this line# include <stdio.h> has the information of what printf will do. Stdio means standard input output and .h represents that it is a header file (as it sticks in the head of the program).
Remember we said, we need to use compilers to translate our language into computers?? There are some compilers available on the internet, GNU GCC compiler is one of the most used one. Download TDM-GCC Compiler from SourceForge.net you can download it from here.
And we need a specialized environment in order to execute the compiler. We call it IDE (Integrated Development Environment). We use code::blocks the most, you can download it from here.. Binary releases - Code::Blocks (codeblocks.org) . It is available for windows, Macintosh OS and Linux.
Now after installing Code::blocks, you will see this..
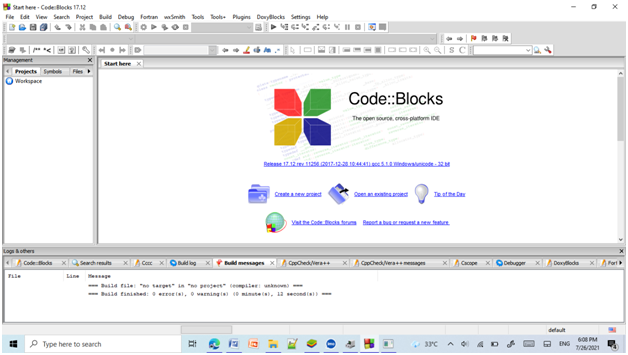
All you have to do is to click Ctrl + Shift + N is windows (Command + Shift +N in macOS) and you will see this..
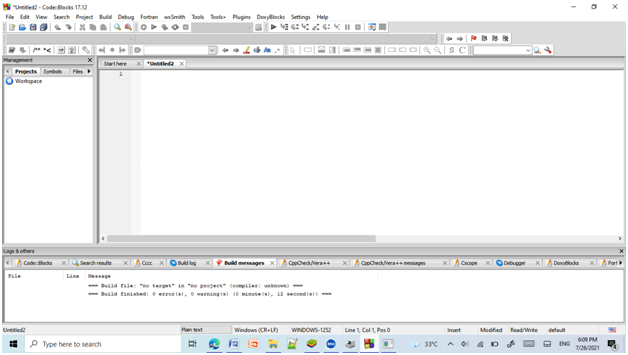
You then have to give it a file extension. Means you have to save this file as .c, then the computer will treat the file as a C source code file.
Simply click Ctrl + S…and this will pop-up:
You have to give your file a name and a .c extension, that’s all!
I named it Myfirstprogram and gave it a .c extension (Myfirstprogram.c). Now the computer will treat the file as a C source code. Click save now.
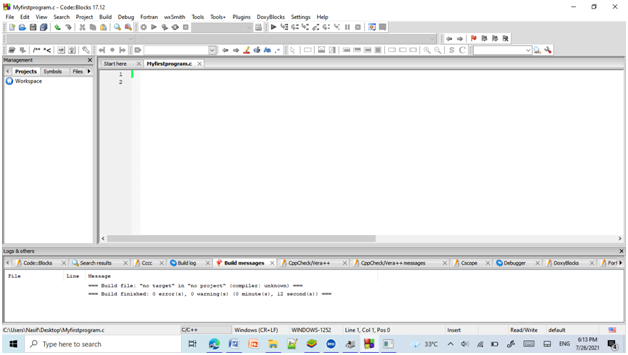
This will appear. Now paste the code I have shown you before here.
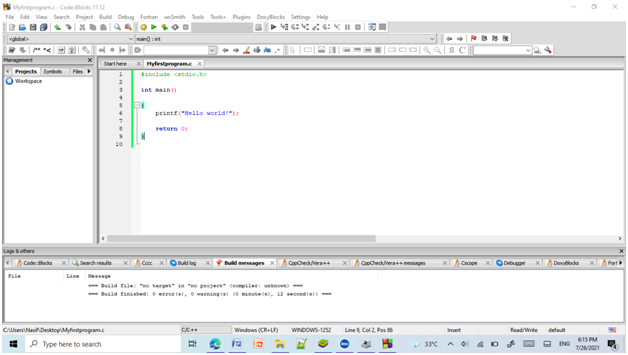
See a play button above??? This button executes your code. But first, you have to translate your code into machine language. You will not do that, the compiler will do it for you, if you click the
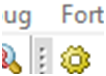
settings (build) button. Then click the play button, you will see this.
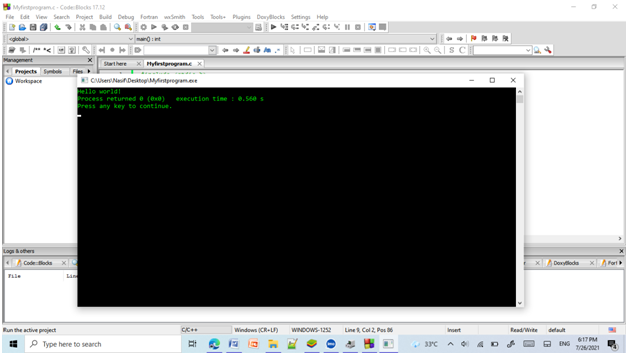
This is your first program! This prints hello world on the screen.
I have explained earlier what does the line # include <stdio.h> do. It has some commands in it, which you can use in your code. And the compiler can translate those into machine language. Like printf, after you have included stdio.h, and you wrote printf, the compiler understood you want to display something on the screen. Likewise, there are other commands in it, which are very handy.
Every car needs a driver. Every application also needs a driver. Int main () is a driver function. In the int main() function, we code what we intent to do with this program. After learning function you will get a vast idea about this.
In the second brackets we command the compiler what we want to do with a function. Like after declaring a function named int main(), we used { } bracket and whatever is written inside it, will be executed.
Return 0; will be described later.
Variables and Data Types
Think of this math problem.
120 + 346 = 466
466 + 120 = 586
586 + 346 = 932.
But if we let a = 120 and b = 346, we can simplify this equation.
a + b = 466
466 + a = 586
586 + b = 932.
If a or b changes, the equation will change, but keep its form intact.
This is a simple idea of algebra, converting any numbers into variables, so work could be done easier.
Variable means “to vary” it can change. When you will make programs, you have to use a lot of numbers or strings, which you will have to assign in some variables. C has several types of variables. Some of them are-
Integers = integer is a number which consist no decimal points. It can be either positive or negative. Defined using int, short, long or long long.
See this example –
See how we declared a variable in c, first we tell the compiler the properties of our number. then we tell the compiler what will be the name of our variable. Then we enter the number. And remember, right now, enter a ; after every line of code which is written in the driver function, or inside the { }.
We assigned 5 as a integer in the variable a, 10 as a short variable in b,
900000 as a long variable in c and 100000000000 as a veryy long variable in d.
this concept is important, c generally lets variables to use a less amount of
memory to execute the code quicker and making the application smaller. But if
you have to use bigger numbers, you have to use long or long long.
Unsigned Integers – They can only be positive. Syntax- unsigned int, unsigned short, unsigned long or unsigned long long.
Floating point numbers – Those are the numbers which consists decimal points. (Number with fractions) Defined using float and double, double uses more memory.
Characters – Those 3 calls only apply for numbers, but c uses a special definition for characters. They are defined using char. See this example..
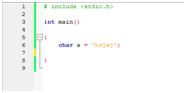
See. We declare a variable named a, and we tell the compiler it will store “A” letter (yes, c allows to insert only one letter in a variable). Then we inserted the variable, but take a look, we used ‘’. This is used to differentiate variable name and the value of the variable.
There is another datatype named Boolean, we will discuss about it later.
Printing variables
Integers are also known as decimals. Note that.
Take a look at this code.
First of all we named a variable a, and assigned an integer in it. Then we decided to print the variable. So we used the printf method, which prints something in the screen. Keep in mind whatever is written in “” in printf, will be printed on the screen. We know integers are known as decimals, by writing printf (“%d”, a); we command the compiler to print a integer, or a decimal, and the name of the decimal is a.
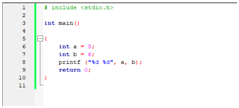
Now take a look at this, we assigned two variables a and b, and printed it.
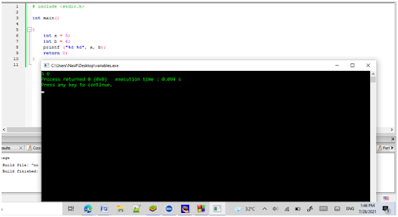
This is the result. I guess you understood, in printf, wherever the %d is, that will be replaced with the value of the variable. And it works respectively, first %d prints the first variable written after the comma. You will clearly understand after this example.
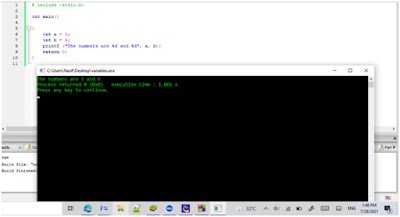
See, here, %d s are replaced, not the other texts. And now you are on your own, I will just tell you what keyword you have to use for other variables. They are also known as format specifiers.
Int = %d
Float = %f
Double = %lf
Char = %c
Short int or unsigned int = %hd
Long int or long unsigned int = %ld
C Input
All real life programs are made with the utility to take input from user right? Now we will learn how to take input in C.
Printf() was used to print something, or make an output. And we use the scanf() method commonly to take input from the user. Stdio.h header file consists the specification of the scanf() method.
Now, lets learn how to take input.
Taking input is pretty easy if you understood the previous part. Just you have to add a &(ampersand) before a variable name after the comma, and you have to declare a variable name and its type before. Watch this,
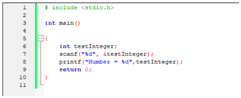
The output is-
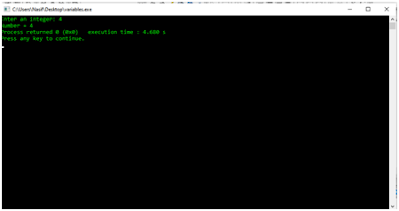
Here, we have used %d format specifier inside the scanf() function to take int (integer) input from the user. When the user enters an integer, it is stored in the testInteger variable.
Notice that we have used &testInteger inside scanf(). That’s because &testInteger gets the address of testInteger, then stores the value which is entered by the user.
Use other format specifiers (%f, %c) and see the results.
Note: If you are using multiple
variables of same type, you can integrate them like- int a, b, c = 3,4,5; it
will assign 3 in a, 4 in b and 5 in c.
Variable naming rules
- A variable name can only have letters (both uppercase and lowercase), digit and underscore.
- The first letter of a variable should be a letter, or an underscore, not a number.
- You can set a variable as large as you want, but you may run into some problems if your variable name is bigger than 31 characters.
- You should always try to give a meaningful name for your variables. For example: firstname is a better variable name than fn.
- Once you declare a variable, you can’t use that name for another variable, or another datatype. That makes C a strongly typed language.
- You can’t use C allocated keywords as your variable name.
C keywords
To successfully compile a code, c predefined some words used in programming that have special meaning to the compiler. Keywords are part of the syntax and cannot be used as an identifier. For example:
int a; here int is a keyword that indicates a is a variable of integer type.
C is a case sensitive language so, all keywords should be written in lowercase. ANSI C recommends the keywords given below-
auto |
double |
int |
struct |
break |
else |
long |
switch |
case |
enum |
register |
typedef |
char |
extern |
return |
union |
continue |
for |
signed |
void |
do |
if |
static |
while |
default |
goto |
sizeof |
volatile |
const |
float |
short |
unsigned |
You will find each and every one of them later.
Types of integers
- Decimal: it has a base of 10. Example: int a = 27;
- Octal: has a base of 8. Example: int b = 021; (note: c takes a number as an octal nimber when a 0 is present before the numbers)
- Hexadecimal: 16 based. Example: int c = 0x7f (Note: hexadecimal should start with 0x)
Escape Sequences
Sometimes it is necessary to use characters that cannot be typed or has a special meaning in C programming. For example, question mark, apostrophe, newline, tab etc. in order to use those, escape sequences were introduced.
For example, \n is used for a new line creation. The syntax is- printf(“Hello world!\nThis is a new line”);
Here, \n will be replaced with an enter.
Other escape sequences are,
\t – tab
\f – Form feed (Goes to the top of a new page)
\r – return to the beginning of the current line
\t - horizontal tab
\v – vertical tab
\\ - backslash(\)
\’ – single quotation mark
\” – double quotation mark
\? – question mark
\0 – null character
The backslash tells the compiler to execute the escape sequences, or they
would have been handled normally by the compiler.
Constants
If you want to define a value that won’t change anymore in the program, you can use the const keyword. This will make the variable a constant.
Example : const
double PI = 3.1416;
This value can’t be changed later in the program.
In the next article, you will learn conditions, loops,arrays and functions in c. Stay connected with us! Thanks.
Post a Comment